Note
Click here to download the full example code
Nifti volumes - GLM analysis¶
Importage¶
# KF Tools and related imports
from kftools.data import fetch_file
from kftools.nifti import ft_glm_ana
import numpy as np
import nibabel as nib
from nilearn.image import mean_img
from nilearn.surface import load_surf_mesh,load_surf_data,vol_to_surf
from nilearn import datasets
from nilearn.plotting import plot_glass_brain,plot_stat_map,plot_surf_stat_map
from matplotlib import pyplot as plt
Out:
/opt/hostedtoolcache/Python/3.8.13/x64/lib/python3.8/site-packages/nilearn/input_data/__init__.py:27: FutureWarning: The import path 'nilearn.input_data' is deprecated in version 0.9. Importing from 'nilearn.input_data' will be possible at least until release 0.13.0. Please import from 'nilearn.maskers' instead.
warnings.warn(message, FutureWarning)
Grab the data¶
data_dir='.'
fetch_file(data_dir=data_dir, filetype='kp-nii-hbo',
site='pitch', task='ft', subid='sub010', sesid='ses01')
fetch_file(data_dir=data_dir, filetype='kp-nii-hbr',
site='pitch', task='ft', subid='sub010', sesid='ses01')
fetch_file(data_dir=data_dir, filetype='kp-nii-evs',
site='pitch', task='ft', subid='sub010', sesid='ses01')
nii_hbo_f = 'pitch_sub010_ft_ses01_1017-1706_kp-nii-hbo.nii.gz'
nii_hbr_f = 'pitch_sub010_ft_ses01_1017-1706_kp-nii-hbr.nii.gz'
nii_ev_f = 'pitch_sub010_ft_ses01_1017-1706_kp-nii-evs.tsv'
Out:
Downloading pitch_sub010_ft_ses01_1017-1706_kp-nii-hbo.nii.gz
Downloading...
From: https://drive.google.com/uc?id=1BdkWzxl4zEKrWmJ79wiWHFImpJ9VVUCk
To: /home/runner/work/kernel-flow-tools/kernel-flow-tools/examples/pitch_sub010_ft_ses01_1017-1706_kp-nii-hbo.nii.gz
0%| | 0.00/63.9M [00:00<?, ?B/s]
3%|3 | 2.10M/63.9M [00:00<00:03, 20.1MB/s]
14%|#3 | 8.91M/63.9M [00:00<00:01, 40.0MB/s]
27%|##7 | 17.3M/63.9M [00:00<00:01, 39.2MB/s]
40%|#### | 25.7M/63.9M [00:00<00:01, 36.3MB/s]
53%|#####3 | 34.1M/63.9M [00:00<00:00, 39.3MB/s]
66%|######6 | 42.5M/63.9M [00:01<00:00, 42.1MB/s]
80%|#######9 | 50.9M/63.9M [00:01<00:00, 41.4MB/s]
93%|#########2| 59.2M/63.9M [00:01<00:00, 41.8MB/s]
100%|##########| 63.9M/63.9M [00:01<00:00, 42.7MB/s]
Downloading pitch_sub010_ft_ses01_1017-1706_kp-nii-hbr.nii.gz
Downloading...
From: https://drive.google.com/uc?id=1BgygbQd3W9GdsSGd4t1TlS6uaPy1_v6l
To: /home/runner/work/kernel-flow-tools/kernel-flow-tools/examples/pitch_sub010_ft_ses01_1017-1706_kp-nii-hbr.nii.gz
0%| | 0.00/63.8M [00:00<?, ?B/s]
2%|1 | 1.05M/63.8M [00:00<00:06, 9.43MB/s]
14%|#3 | 8.91M/63.8M [00:00<00:01, 35.0MB/s]
20%|#9 | 12.6M/63.8M [00:00<00:01, 26.7MB/s]
29%|##8 | 18.4M/63.8M [00:00<00:01, 30.2MB/s]
41%|####1 | 26.2M/63.8M [00:00<00:01, 32.6MB/s]
53%|#####3 | 34.1M/63.8M [00:01<00:00, 30.2MB/s]
67%|######6 | 42.5M/63.8M [00:01<00:00, 31.9MB/s]
80%|#######9 | 50.9M/63.8M [00:01<00:00, 29.8MB/s]
93%|#########2| 59.2M/63.8M [00:01<00:00, 35.1MB/s]
100%|##########| 63.8M/63.8M [00:01<00:00, 34.1MB/s]
Downloading pitch_sub010_ft_ses01_1017-1706_kp-nii-evs.tsv
Downloading...
From: https://drive.google.com/uc?id=1BSLMnD5Hmkj3g_YfKioLQUL3AQfvV5ac
To: /home/runner/work/kernel-flow-tools/kernel-flow-tools/examples/pitch_sub010_ft_ses01_1017-1706_kp-nii-evs.tsv
0%| | 0.00/47.2k [00:00<?, ?B/s]
100%|##########| 47.2k/47.2k [00:00<00:00, 10.6MB/s]
Analysis and viz¶
Run GLM analysis
res_hbo = ft_glm_ana(nii_hbo_f, nii_ev_f, out_fstr = '')
z_thrs,glm,evs,cntrsts,img = res_hbo
z = z_thrs['rightft_minus_rest']
Out:
/home/runner/work/kernel-flow-tools/kernel-flow-tools/kftools/nifti/glm.py:38: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
evs['trial_type'][evs.block_type=='left'] = 'left'
/home/runner/work/kernel-flow-tools/kernel-flow-tools/kftools/nifti/glm.py:39: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
evs['trial_type'][evs.block_type=='right'] = 'right'
/opt/hostedtoolcache/Python/3.8.13/x64/lib/python3.8/site-packages/nilearn/glm/first_level/first_level.py:64: UserWarning: Mean values of 0 observed.The data have probably been centered.Scaling might not work as expected
warn('Mean values of 0 observed.'
Glass brain plots
disp = plot_glass_brain(z,colorbar=True,threshold=5,black_bg=True)
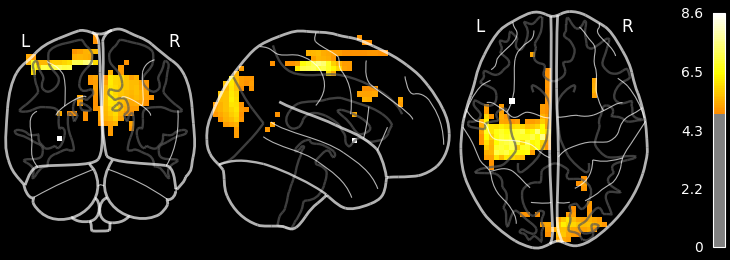
Slice view stat image plots
disp = plot_stat_map(z,colorbar=True,threshold=5,cut_coords=[-20,-20,60])
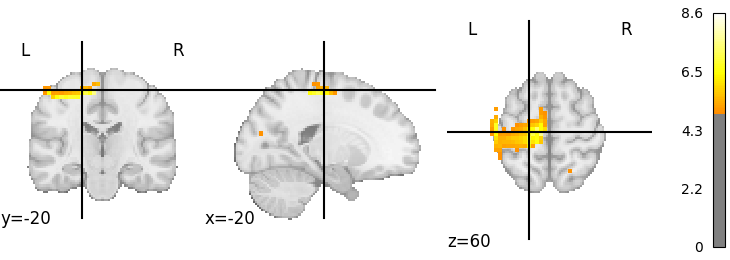
Project to surface
fs5 = datasets.fetch_surf_fsaverage()
lh_dat = vol_to_surf(z,surf_mesh=fs5.pial_left)
rh_dat = vol_to_surf(z,surf_mesh=fs5.pial_right)
lhc = load_surf_data(fs5.sulc_left)
rhc = load_surf_data(fs5.sulc_right)
lhi_vtx,lhi_tri = load_surf_mesh(fs5.infl_left)
rhi_vtx,rhi_tri = load_surf_mesh(fs5.infl_right)
rhi_vtx_mod = rhi_vtx.copy()
rhi_vtx_mod[:,0] += 90
lrhi_vtx = np.concatenate([lhi_vtx, rhi_vtx_mod],axis=0)
lrhi_tri = np.concatenate([lhi_tri, rhi_tri+lhi_vtx.shape[0]])
lrh_dat = np.concatenate([lh_dat,rh_dat],axis=0)
lrhc = np.concatenate([lhc,rhc],axis=0)
lrhi_vtx_rot = np.zeros_like(lrhi_vtx)
lrhi_vtx_rot[:,0] = -lrhi_vtx[:,1]
lrhi_vtx_rot[:,1] = lrhi_vtx[:,0]
lrhi_vtx_rot[:,2] = lrhi_vtx[:,2]
fig, ax = plt.subplots(ncols=3,subplot_kw={'projection': '3d'},figsize=(8,3))#, f)
a = ax[0]
disp = plot_surf_stat_map([lrhi_vtx_rot,lrhi_tri],lrh_dat, hemi='right', view='dorsal',
bg_map=lrhc,threshold=5,axes=a,colorbar=False)#
a = ax[1]
disp = plot_surf_stat_map([lhi_vtx,lhi_tri],lh_dat, hemi='left', view='lateral',
bg_map=lhc,threshold=5,axes=a,colorbar=False)#
a = ax[2]
disp = plot_surf_stat_map([rhi_vtx,rhi_tri],rh_dat, hemi='right', view='lateral',
bg_map=rhc,threshold=5,axes=a,colorbar=False)#
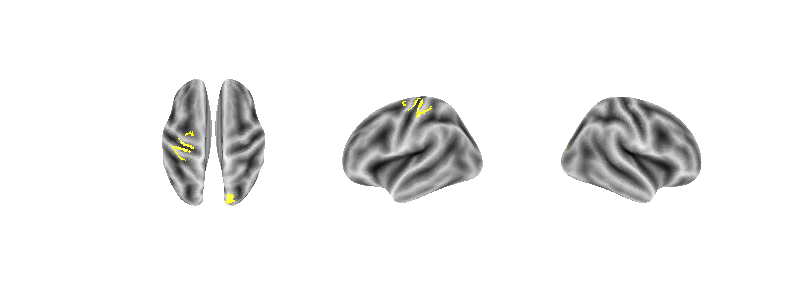
Total running time of the script: ( 0 minutes 24.833 seconds)